search
Unleashing Tech Brilliance: Embrace Inclusivity, Ignite Diversity! Join our vibrant community, propelling women, minorities, and tech enthusiasts towards boundless possibilities. Together, we break barriers, shatter stereotypes, and champion empowerment. Dive into a world of support, inspiration, and cutting-edge insights, as we revolutionize tech - one diverse mind at a time!
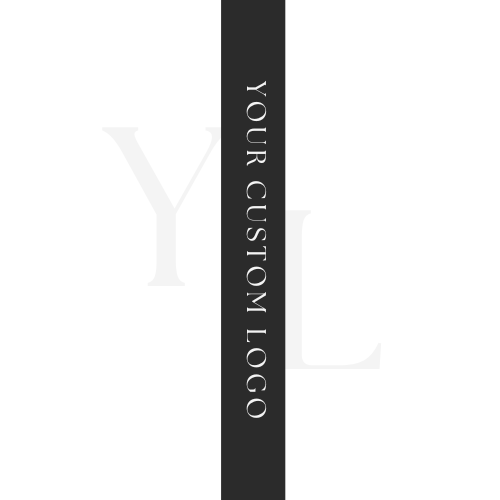
Adriana Lamb
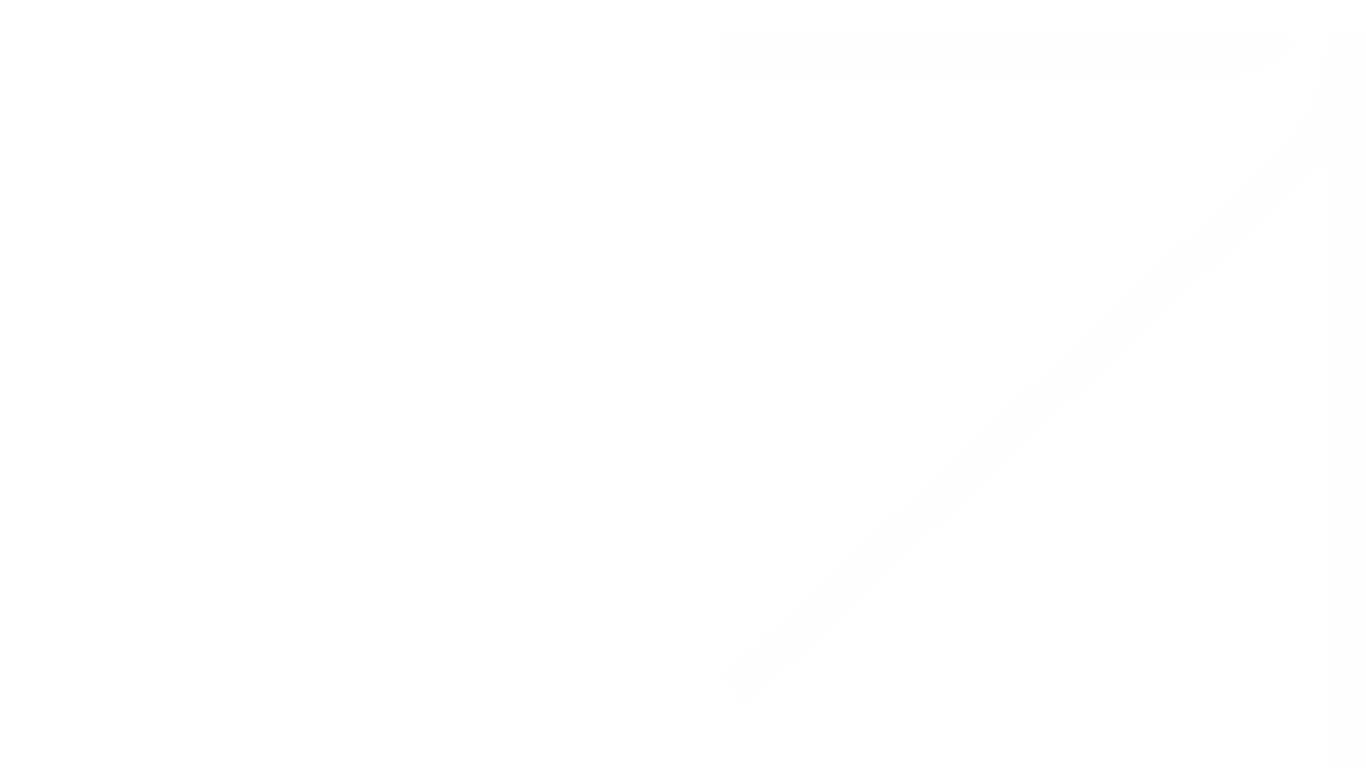.png)
Hi, I'm
Adriana Lamb +
Welcome to my website
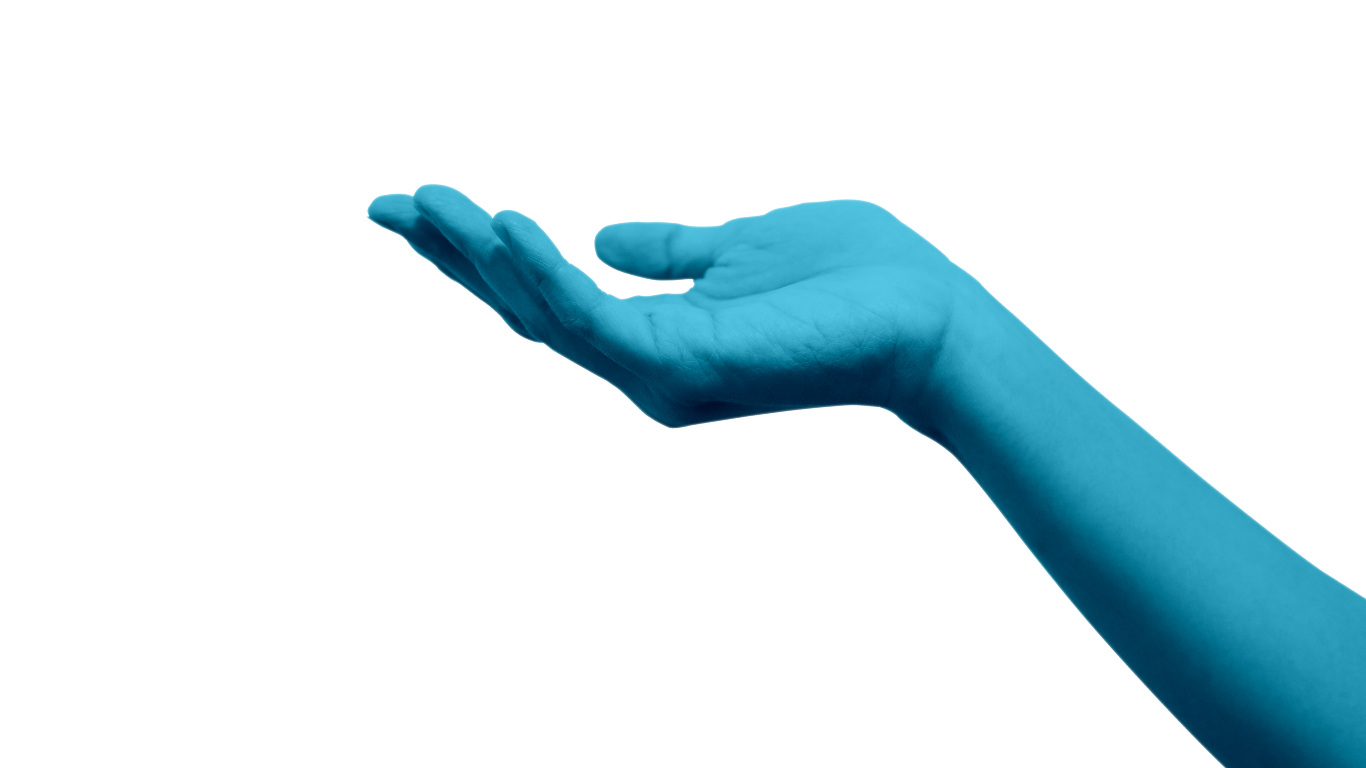.png)
.png)
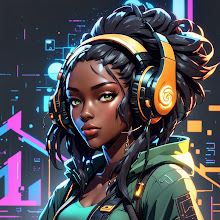
A Little About Me...
I am a an experienced full-stack developer specializing in cybersecurity, AWS cloud engineering, Angular, and big data development. My expertise is complemented by a drive for continuous improvement. Attending various seminars and boot camps, my focus is on honing skills and enhancing my capabilities in coding and software development.
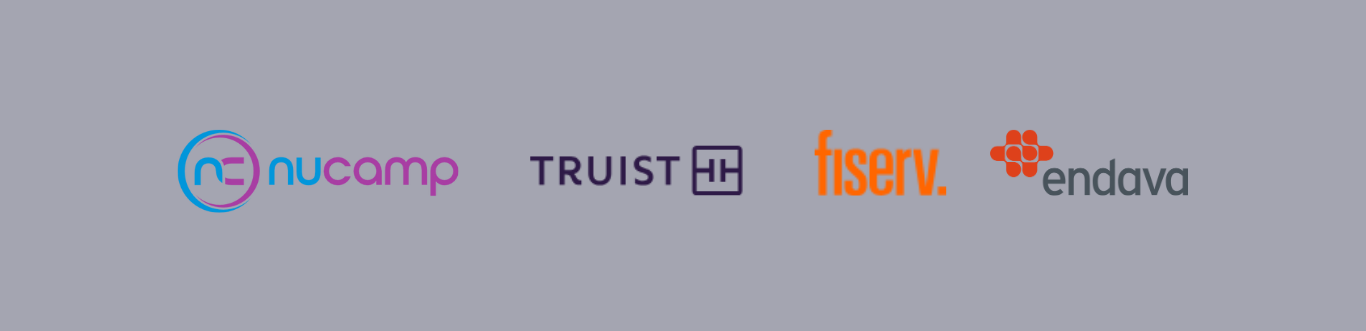.png)
Innovation That Flows
.png)
Full-Stack developer
I offer Full-Stack Development expertise, blending front-end finesse with back-end efficiency. Skilled in HTML, CSS, JavaScript, Python, Node.js, and Java, I create intuitive interfaces and robust back-end solutions for optimized user experiences.
Responsive Designs
I specialize in comprehensive Full-Stack Development, seamlessly integrating front-end finesse with back-end efficiency. With expertise in HTML, CSS, and JavaScript, I craft intuitive interfaces that elevate user experiences.
Mentorship
As your programming mentor, I'm here to supercharge your skills. Through dynamic lessons and exciting projects, I'll propel your coding journey forward. Let's unleash your full potential and create amazing things together!
"In a world of algorithms and lines of code, remember that YOU are the true source of innovation."
I approach my work as a full-stack developer with enthusiasm, curiosity, and a drive to achieve excellence. Over 5 rewarding years in technology consulting, I have been fortunate enough to serve clients around the world, advise startups, and play an instrumental role in some of the most cutting-edge digital innovation projects. With vast experience in cybersecurity, cloud engineering, front-end design, and big data solutions, I am thrilled to constantly be learning and honing my skills for the sake of delivering outstanding results for my clients. Technology is empowering me to make a real impact through inspired design decisions!
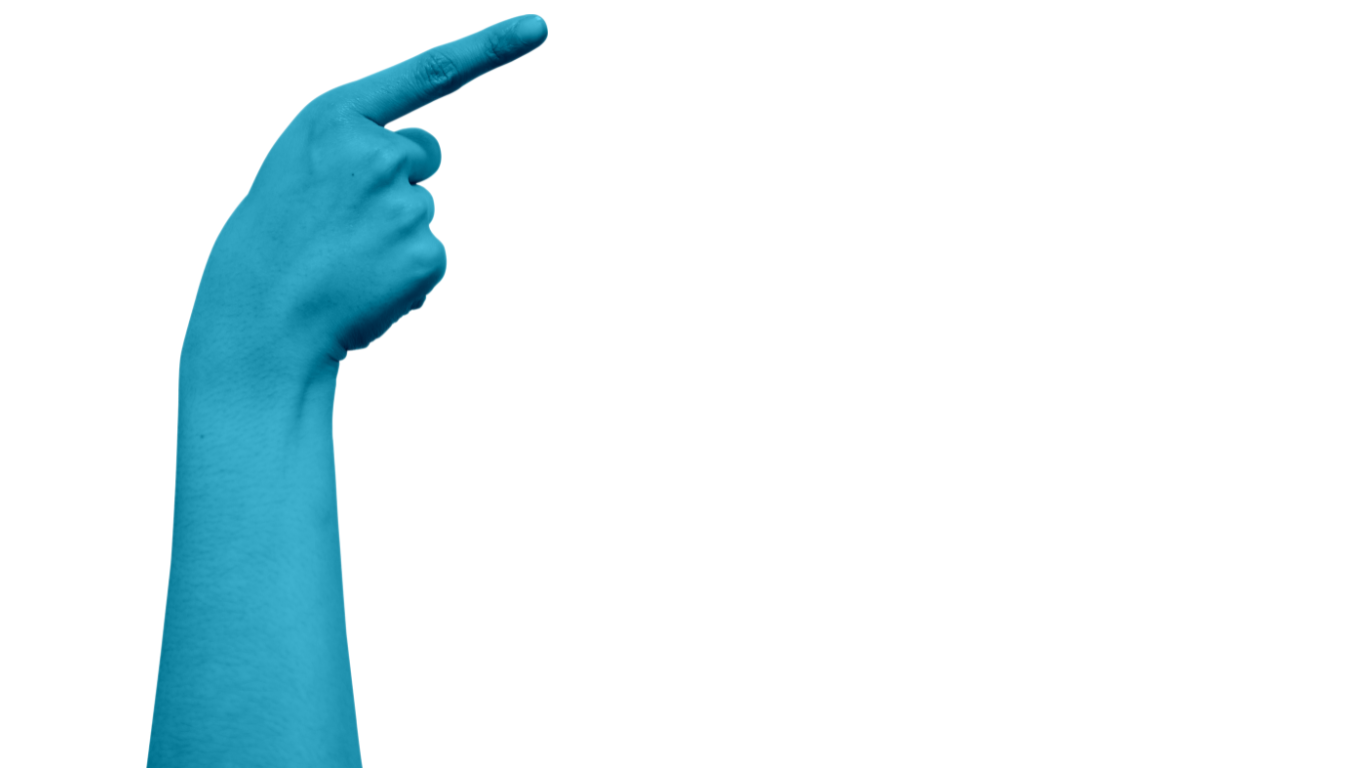.png)
- Angular
- React
- Django
- Bootstrap
- JavaScript
- Node.js
- Typescript
Front-End Development
- Java
- Go
- SQL
- Spark
- Posgres
- DynamoDB
- C#
- C++
- Python
- Maven
- Gradle
Back-End Development
- AWS
- GoCD
- Jenkins
- Terraform
- Veracode
- Nexus
- Sonatype
- WinSCP
- Putty
- Linux
- Makefile
- Bash
DevOps
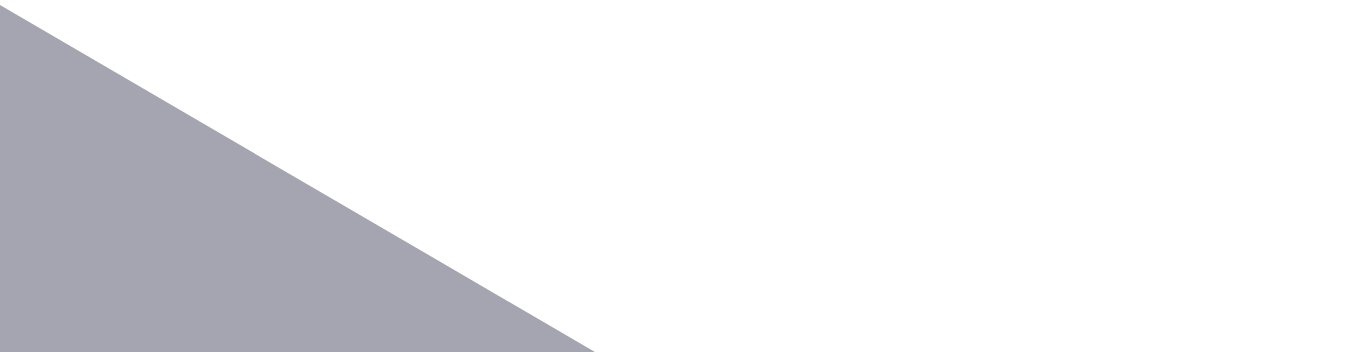.png)
Let's Talk!
Have any project ideas? Looking to kickstart your career in tech? Schedule time with me to talk!
Tech spans beyond code, driving innovation in design, science, healthcare, and more.
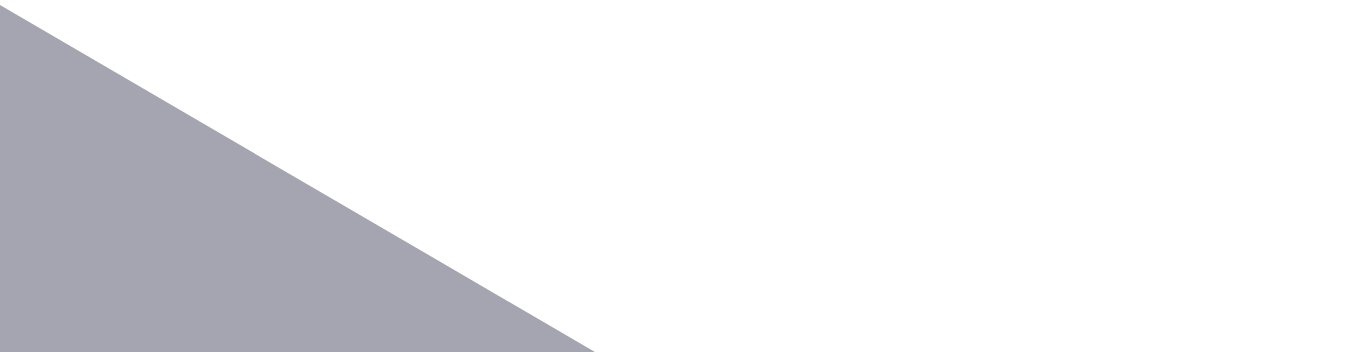.png)
Psst...I'm growing a community specifically focused on teaching YOU more about programming.
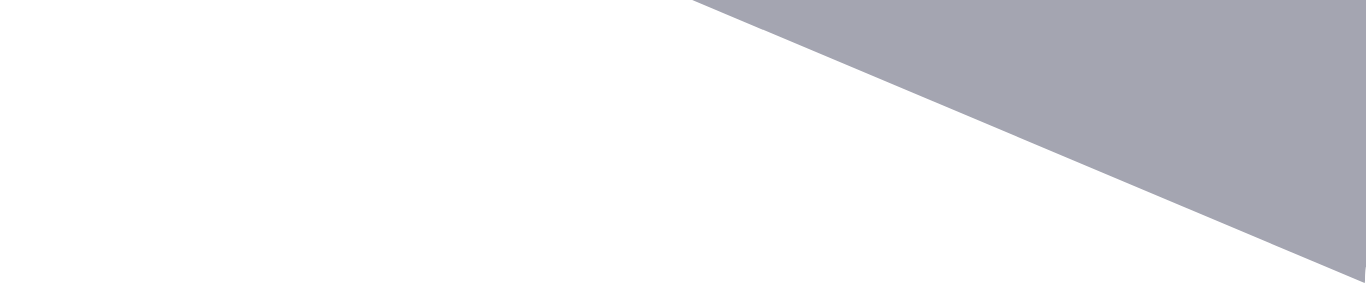.png)
Featured
- Get link
- X
- Other Apps
A Step-by-Step Guide for Building a Bot with Go (Part 1)
package main
func main() {
}
module bubble.go
go 1.17
go get -u github.com/tebeka/selenium
go get -u github.com/tebeka/selenium/chrome
import (
"github.com/tebeka/selenium"
"github.com/tebeka/selenium/chrome"
)
func main() {
//create a webdriver instance
driver, err := selenium.NewChromeDriverService("./chromedriver",4444)
if err != nil {
panic(err)
}
defer driver.Stop()
//add custom driver capabilities as a chrome driver
caps := selenium.Capabilities{}
caps.AddChrome(chrome.Capabilities{Args: []string{
"window-size=1920x1080",
}})
//create a new remote client with configured capabilities
wd, err := selenium.NewRemote(caps, "")
if err != nil {
panic(err)
}
//tells the driver to go to desired webpage
wd.Get("[WHATEVERWEBSITEYOUCHOOSE]")
}
func login(wd selenium.WebDriver) error {
wd.SetImplicitWaitTimeout(60000000000 * time.Nanosecond)
loginBtn, err := wd.FindElement(selenium.ByXPATH, "//location of login btn")
if err != nil {
return err
}
if err = loginBtn.Click(); err != nil {
return err
}
email, err := wd.FindElement(selenium.ByXPATH, "//*[@id=\"email\"]")
if err != nil {
return err
}
password, err := wd.FindElement(selenium.ByXPATH, "//*[@id=\"password\"]")
if err != nil {
return err
}
if err = email.SendKeys("your email address"); err != nil {
return err
}
if err = password.SendKeys("your password"); err != nil {
return err
}
loginBtn, err = wd.FindElement(selenium.ByXPATH, "//this is the second login btn")
if err = loginBtn.Click(); err != nil {
return err
}
fmt.Println("We're logged in!!!")
return nil
}
func search(wd selenium.WebDriver) error {
wd.SetImplicitWaitTimeout(60000000000)
searchBar, err := wd.FindElement(selenium.ByXPATH, "//location of search box")
if err != nil {
return err
}
searchBar.SendKeys("stuff\n")
fmt.Printf("We were able to search")
return err
}
func scrollForElement(wd selenium.WebDriver, parentXpath string, xpath string) error {
var err error
script := fmt.Sprintf(`
var elem = document.evaluate("%s", parent, null, XPathResult.FIRST_ORDERED_NODE_TYPE, null).singleNodeValue;
var parent = document.evaluate("%s", document, null, XPathResult.FIRST_ORDERED_NODE_TYPE, null).singleNodeValue;
if (elem) {
elem.click();
return true;
} else {
parent.scrollTop = parent.scrollHeight;
return false;
}
`, parentXpath, xpath)
found := false
for !found {
if _, err := wd.ExecuteScript(script, nil); err != nil {
return fmt.Errorf("failed to execute script: %w", err)
}
elem, err := wd.FindElement(selenium.ByXPATH, xpath)
if elem != nil {
found = true
}
if err != nil {
return errors.New("Failed to find element")
}
}
return err
}
func savePost(wd selenium.WebDriver, err error) error {
saveButton := "//*save button location"
err = scrollForElement(wd, saveButton, "//page")
if err != nil {
fmt.Println(err.Error())
return err
}
wd.Back()
fmt.Println("Saved the element going to previous page")
return nil // no error
}
func getPosts(wd selenium.WebDriver) error {
var wg sync.WaitGroup
div := 1
var err error
for i := 1; i < 10; i++ {
wg.Add(1)
go func(i int) {
defer wg.Done()
xpath := fmt.Sprintf("//post location", div)
_, err := wd.FindElement(selenium.ByXPATH, xpath)
for err != nil {
div++
xpath := fmt.Sprintf("//xpath with an increased div count in case there is an ad", div)
err = scrollForElement(wd, xpath, "//page")
if err != nil {
return
}
err = savePost(wd, err)
if err != nil {
return
}
fmt.Printf("\nWe have successfully saved a post %d", i)
div++
}else{
post, err := wd.FindElement(selenium.ByXPATH, fmt.Sprintf("//post", div)
post.Click()
err = savePost(wd, err)
if err != nil {
return
} else {
fmt.Printf("\nWe have successfully saved a post %d", i)
div++
}
}
}(i)
}
wg.Wait()
fmt.Println("\nI think this works")
return err
}
import (
"errors"
"fmt"
"sync"
"time"
"github.com/tebeka/selenium"
"github.com/tebeka/selenium/chrome"
)
func main() {
driver, err := selenium.NewChromeDriverService("./chromedriver", 4444)
if err != nil {
panic(err)
}
defer driver.Stop()
caps := selenium.Capabilities{}
caps.AddChrome(chrome.Capabilities{Args: []string{
"window-size=1920x1080",
}})
wd, err := selenium.NewRemote(caps, "")
if err != nil {
panic(err)
}
wd.DeleteAllCookies()
if err := wd.Get("[Website]"); err != nil {
fmt.Printf("Could not log into site: %s", err)
}
if err = login(wd); err != nil {
fmt.Printf("Could not log into site: %s", err)
}
if err = search(wd); err != nil {
fmt.Printf("Unable to submit search: %s", err)
}
if err = getPosts(wd); err != nil {
fmt.Printf("Failed to get Posts: %s", err)
}
}
Sure, there are more features we could add, but that'll be your job to explore. The second section of this guide will involve us setting up a more official bot that will require you to utilize a popular social media platform's API.
- Get link
- X
- Other Apps
Comments
Post a Comment